이롭게 현명하게
[JavaScript] 배열 내장 함수 본문
목차
forEach
map
filter
splice와 slice
shift, pop, unshift, push
reduce
[ forEach ]
배열 안에 있는 모든 원소들을 출력하고 싶을 때 사용한다.
const coding = [
"c",
"Java",
"JavaScript",
"Python",
]
console.log(coding) // ["c", "Java", "JavaScript", "Python"]
// 반복문 사용
for (let i = 0; i<coding.length;i++){
console.log(coding[i])
}
// 함수
function printcoding(lang){
console.log(lang)
}
// forEach문으로 출력
coding.forEach(printcoding)
// 익명함수를 통해 forEach문으로 출력
coding.forEach(function (lang){
console.log(lang)
})
// 화살표 함수를 통해 forEach문으로 출력
coding.forEach((lang)=>{
console.log(lang)
})
//c
//Java
//JavaScript
//Python
// 에러
coding.forEach()
[ map ]
배열 안에 모든 원소를 변환할 때 사용
for, forEach문을 사용해서 원소를 변환할 수 있지만
map이 보다 더 간편하다
arr = [1,2,3,4,5,6,7,8,9]가 있다.
이 arr를 2의 배수인 배열 tmp를 만들려면 for문 혹은 forEach문을 사용해야 한다.
tmp1은 2의 배수로, tmp2는 3의 배수로 만들었다.
const arr = [1,2,3,4,5,6,7,8,9]
const tmp1 = []
const tmp2 = []
//for문 사용
for (let i = 0;i<arr.length;i++){
tmp1.push(arr[i]*2)
}
//forEach문 사용
arr.forEach(n=>{
tmp2.push(n*3)
})
console.log(tmp1) // [2, 4, 6, 8, 10, 12, 14, 16, 18]
console.log(tmp2) // [3, 6, 9, 12, 15, 18, 21, 24, 27]
하지만 map을 사용하면 코드가 간결해진다.
const arr = [1,2,3,4,5,6,7,8,9]
const mul1 = n => n*2
const mul2 = n => n*3
const tmp1 = arr.map(mul1)
const tmp2 = arr.map(n => n*3)
console.log(tmp1) // [2, 4, 6, 8, 10, 12, 14, 16, 18]
console.log(tmp2) // [3, 6, 9, 12, 15, 18, 21, 24, 27]
monster라는 객체가 있다.
const monster =[
{
name:'monster',
HP:100,
defense:50
},
{
name:'zombie',
HP:100,
defense:30
}
]
객체 배열(monster)을 문자열로 이루어진 배열로 만들 수 있다.
const monster =[
{
name:'monster',
HP:100,
defense:50
},
{
name:'zombie',
HP:100,
defense:30
}
]
const arr = monster.map(n => n.name)
console.log(arr) // ["monster", "zombie"]
배열 coding에서 "JavaScript" 원소의 위치를 알고 싶다면 indexOf 함수를 사용한다.
const coding = [
"c",
"Java",
"JavaScript",
"Python",
]
const index = coding.indexOf("JavaScript")
console.log(index) // 2
배열에서 원하는 항목이 몇 번째 원소인지 알려주는 함수 : indexOf
배열 안에 있는 값이 문자열이나 숫자 혹은 boolean이면 찾고자 하는 원소가 몇 번째에 있는지 indexOf 함수만으로도 충분히 찾을 수 있다.
하지만 만약 배열 안에 있는 값들이 객체이거나 특정값이 일치하는 것이 아닌 어떠한 조건을 찾는다면 indexOf로는 불가능하다.
배열 안에 있는 값들이 객체이거나 특정값이 일치하는 것이 아닌 어떠한 조건을 찾을 때는 findeindex함수를 사용한다.
const todos = [
{
id : 1,
text : "자바스크립트 입문",
done : true
},
{
id : 2,
text : "함수 배우기",
done : true
},
{
id : 3,
text : "객체와 배열 배우기",
done : true
},
{
id : 4,
text : "배열 내장함수 배우기",
done : false
}
]
//id가 3인 객체
const index = todos.indexOf(3)
console.log(index) // -1 : 일치하는 것이 없다.
const findex = todos.findIndex(todo => todo.id ===3)
console.log(findex) // 2 : 배열 2번 인덱스에 있다.
find 함수는 객체 자체 혹은 원소를 찾아 객체를 반환한다.
const todos = [
{
id : 1,
text : "자바스크립트 입문",
done : true
},
{
id : 2,
text : "함수 배우기",
done : true
},
{
id : 3,
text : "객체와 배열 배우기",
done : true
},
{
id : 4,
text : "배열 내장함수 배우기",
done : false
}
]
const findex = todos.find(todo => todo.id ===3)
console.log(findex) // {id: 3, text: "객체와 배열 배우기", done: true}
[ filter ]
특정 조건을 만족하는 원소들을 찾아서 배열을 만드는 것
const todos = [
{
id : 1,
text : "자바스크립트 입문",
done : true
},
{
id : 2,
text : "함수 배우기",
done : true
},
{
id : 3,
text : "객체와 배열 배우기",
done : true
},
{
id : 4,
text : "배열 내장함수 배우기",
done : false
}
]
const taskNotDone = todos.filter(td => td.done === false)
// 또는 const taskNotDone = todos.filter(td => !td.done)
console.log(taskNotDone) // [Object]
객체들 중에서 done이 false인 값만 나타나게 된다.
[ splice와 slice ]
splice : 배열에서 특정 항목을 제거할 때 사용한다.
제거를 하게 되는 과정에서 제거할 원소가 몇 번째인지 알아야 한다.
인덱스부터 n번째 까지 인덱스를 제거하겠다.
const numbers =[10,20,30,40]
const index = numbers.indexOf(30)
const spliced = numbers.splice(index,2)
console.log(spliced) // [30, 40]
console.log(numbers) // [10, 20]
slice : 배열을 잘라낼 때 사용한다.
splice와의 차이점은 기존의 배열을 건드리지 않고, 파라미터가 다르다.
const numbers =[10,20,30,40]
const sliced = numbers.slice(0,2)
// .slice(start,end)
//start : 자를 인덱스 시작점
// end : end값의 인덱스의 전까지 자른다.
console.log(sliced) // [10, 20]
console.log(numbers) // [10, 20, 30, 40]
[ shift, pop, unshift, push ]
shift : 첫 번째 원소를 배열에서 추출한다.
const numbers =[10,20,30,40]
const value = numbers.shift();
console.log(value) // 10
console.log(numbers) // [20, 30, 40]
pop : 마지막 원소를 배열에서 추출한다.
const numbers =[10,20,30,40]
const value = numbers.pop();
console.log(value) // 40
console.log(numbers) // [10, 20, 30]
unshift : 배열의 첫 번째 원소를 추가
const numbers =[10,20,30,40]
const value = numbers.unshift(5)
console.log(value) // 5
console.log(numbers) // [5, 10, 20, 30, 40]
push : 배열의 마지막에 원소를 추가
const numbers =[10,20,30,40]
const value = numbers.push(5)
console.log(value) // 5
console.log(numbers) // [10, 20, 30, 40, 5]
concat : 여러 개의 배열을 하나의 배열로 합쳐준다.
기존의 배열은 수정되지 않는다.
const arr1 = [1,2,3]
const arr2 = [4,5,6]
const concated = arr1.concat(arr2)
// 또는 const concated = [...arr1,...arr2]
console.log(concated) // [1, 2, 3, 4, 5, 6]
console.log(arr1) // [1, 2, 3]
console.log(arr2) // [4, 5, 6]
join : 배열 안에 있는 값들을 문자열로 합쳐준다.
const arr = [1,2,3,4,5]
console.log(arr.join()) // 1,2,3,4,5
console.log(arr.join('')) // 12345
console.log(arr.join(' ')) // 1 2 3 4 5
console.log(arr.join(', ')) // 1, 2, 3, 4, 5
[ reduce ]
reduce : 배열 안에 있는 값들을 연산해야할 때 사용한다.
배열안에 있는 원소들의 값의 합을 구하려면 다음과 같다.
const numbers =[1,2,3,4,5]
let sum=0
numbers.forEach(n=>{
sum +=n
})
console.log(sum) // 15
reduce 함수를 사용하면 코드가 간결해진다.
const numbers =[1,2,3,4,5]
//(누적된 값, 각 원소들)=>실행할 문장,accumulator 초기값
const sum = numbers.reduce((accumulator,current)=>accumulator+current,0)
console.log(sum) // 15
/*
(누적된 값, 각 원소들,원소 인덱스,실행하고있는 함수의 배열 자신)=>{
실행할 문장
},accumulator 초기값)
*/
const avg = numbers.reduce((accumulator,current,index,array)=>{
if (index === array.length -1){ // 현재 처리하고있는 배열원소가 맨 마지막이라면
return (accumulator + current)/ array.length
}
return accumulator+current
},0)
console.log(avg) // 3
reduce를 사용해 원소의 개수를 구할 수 있다.
const alp = ['a','a','a','b','b','c','d']
const counts = alp.reduce((acc,current)=>{
if (acc[current]){ // acc안에 cur이 있는지 확인
acc[current]+=1
}
else{
acc[current] = 1
}
return acc
},{})
//{} : 비어있는 객체acc
console.log(counts) // {a: 3, b: 2, c: 1, d: 1}
/*
a: 3
b: 2
c: 1
d: 1
*/
잘못된 정보는 댓글에 남겨주시면 감사하겠습니다!😊
댓글과 좋아요는 큰 힘이 됩니다!
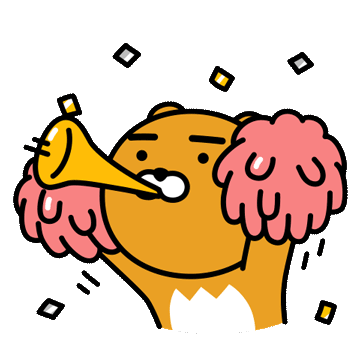
[ 참고자료 ]
'웹 개발 > JavaScript' 카테고리의 다른 글
[JavaScript] 자바스크립트 시작하기 / 개발환경 (1) | 2023.11.27 |
---|---|
[JavaScript] 자바스크립트 시작하기 (1) | 2023.11.24 |
[JavaScript] 자바스크립트 Scope와 hoisting (0) | 2023.05.18 |
[JavaScript] 자바스크립트 spread와 rest (0) | 2023.05.17 |
[JavaScript] 자바스크립트 프로토타입과 클래스 (0) | 2023.05.16 |