이롭게 현명하게
[JavaScript] 자바스크립트 프로토타입과 클래스 본문
목차
프로토타입과 클래스
객체 생성자
객체 생성자 상속하기
ES6 Class
[객체 생성자]
객체 생성자는 함수를 통해서 새로운 객체를 만들고 그 안에 넣고 싶은 값, 또는 함수를 구현할 수 있게 해 준다.
새로운 객체를 만들 때는 new라는 키워드를 사용한다.
function Animal(type, name,sound){
this.type = type;
this.name = name;
this.sound = sound;
this.say = function(){
console.log(this.sound);
}
}
const dog = new Animal('개','멍멍이','멍멍');
const cat = new Animal('고양이','야옹이','야옹');
dog.say();
cat.say();
dog와 cat 객체를 생성하면 type과 name, sound는 각각 다른 값을 가지고 있지만 say라는 함수는 똑같은 내용을 가지고 두 번이나 선언이 되고 있다.(dog생성 시 1번 , cat 생성 시 1번)
say라는 함수의 기능은 똑같은 내용을 가지고 있기 때문에 객체 밖으로 꺼내어 재사용하게 할 수 있다.
이럴 때 사용하는 것이 프로토타입이다.
프로토타입의 역할 : 객체 생성자로 객체를 만들었을 때 만든 객체들끼리 공유할 수 있는 어떠한 값이나 함수를 설정하는 것
function Animal(type, name,sound){
this.type = type;
this.name = name;
this.sound = sound;
}
Animal.prototype.say = function(){
console.log(this.sound);
}
Animal.prototype.sharedValue = 1;
const dog = new Animal('개','멍멍이','멍멍');
const cat = new Animal('고양이','야옹이','야옹');
dog.say();
cat.say();
dog.sharedValue
[객체 생성자 상속하기]
객체 생성자 : 함수를 new 키워드로 사용하여 호출하였을 때 어떠한 새로운 객체를 만들어 기능을 구현할 수 있도록 하는
Cat과 Dog 객체 생성자를 통해 cat과 dog 객체를 생성하였다.
거의 똑같은 내용으로 두 번이나 만들었다.
코드가 중복되면 유지보수가 어려워져 많은 시간과 노력이 필요해집니다.
function Dog(name,sound){
this.type = '개';
this.name = name;
this.sound = sound;
}
function Cat(name,sound){
this.type = '고양이';
this.name = name;
this.sound = sound;
}
Dog.prototype.say = function(){
console.log(this.sound);
}
Cat.prototype.say = function(){
console.log(this.sound);
}
const dog = new Dog('멍멍이','멍멍');
const cat = new Cat('야옹이','야옹');
이러한 코드 중복을 피하기위해 상속을 합니다.
Animal이란 객체생성자를 만들었고 prototype의 say함수를 만들어주었다.
Dog라는 객체 생성자와 Cat이라는 객체 생성자를 만들어 prototype으로 공유하게 하였다.
Animal.call()에서 call()이란 함수는? 첫 번째 파라미터에 이 객체생성자 함수에서의 this를 넣어주고 그 뒤에 파라미터들은 Animal 객체 생성자의 파라미터들을 의미한다.
function Animal(type, name,sound){
this.type = type;
this.name = name;
this.sound = sound;
}
Animal.prototype.say = function(){
console.log(this.sound);
}
function Dog(name,sound){
Animal.call(this,'개',name,sound);
}
function Cat(name,sound){
Animal.call(this,'고양이',name,sound);
}
Dog.prototype = Animal.prototype;
Cat.prototype = Animal.prototype;
const dog = new Dog('멍멍이','멍멍');
const cat = new Cat('야옹이','야옹');
dog.say();
cat.say();
dog.sharedValue
[ES6 Class]
클래스는 C++,JAVA 등의 프로그래밍 언어에는 있는 기능이지만 자바스크립트에서는 없는 기능이다.
자바스크립트 ES6에서는 class가 도입이 되었다.
다른 언어에서의 class와 완전 동일하지는 않다.
class Animal{
constructor (type,name,sound){//생성자
this.type = type;
this.name = name;
this.sound = sound;
}
say(){
console.log(this.sound);
}
}
const dog = new Animal('개','멍멍이','멍멍');
const cat = new Animal('고양이','냥냥이','야옹');
dog.say();
cat.say();
say라는 함수를 class 내부에 구현하였다.
함수를 만들게되면 자동으로 prototype으로 등록이 된다.
만약 console.log(Animal.prototype.say); 를 한다면 say함수가 설정되어 있는 것을 볼 수 있다.
클래스 문법을 사용한다면 상속을 해야하는 상황에서 훨씬 더 쉽게 할 수 있다.
class Animal{
constructor (type,name,sound){//생성자
this.type = type;
this.name = name;
this.sound = sound;
}
say(){
console.log(this.sound);
}
}
class Dog extends Animal{//extends : 특정 클래스를 상속받는다.
constructor(name, sound){ // Animal에서 사용하는 constructor를 덮어쓴다.
//super 키워드를 사용하여 Animal이 가지고있는 constructor을 먼저 호출을 하고 나서
//자신이 해야할 일을 처리할 수 있다.
super('개',name,sound);
}
}
class Cat extends Animal{
constructor(name,sound){
super('고양이',name,sound);
}
}
const dog = new Animal('개','멍멍이','멍멍');
const cat = new Animal('고양이','냥냥이','야옹');
const dog1 = new Dog('댕댕이','왈왈');
const cat1 = new Cat('야옹이','냐옹');
dog.say(); //멍멍
cat.say(); //야옹
cat2.say(); //냐옹
[JavaScript] 자바스크립트 spread와 rest
목차 spread 연산자 rest 함수 파라미터에서의 rest 함수 인자에서의 spread [spread 연산자] spread : 펼치다, 퍼트리다 이 문법을 사용하면 객체 혹은 배열을 펼칠 수 있다. const slime = { name : '슬라임' }; con
devyihyun.tistory.com
잘못된 정보는 댓글에 남겨주시면 감사하겠습니다!😊
댓글과 좋아요는 큰 힘이 됩니다!
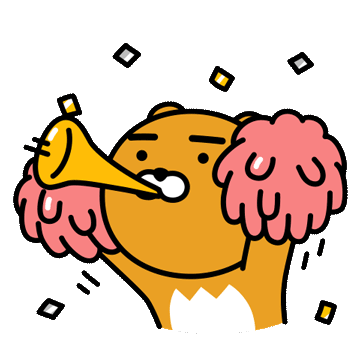
[ 참고자료 ]
'웹 개발 > JavaScript' 카테고리의 다른 글
[JavaScript] 자바스크립트 Scope와 hoisting (0) | 2023.05.18 |
---|---|
[JavaScript] 자바스크립트 spread와 rest (0) | 2023.05.17 |
[JavaScript] 자바스크립트 객체 (0) | 2023.05.15 |
[JavaScript] 자바스크립트 화살표 함수 (0) | 2023.05.12 |
[JavaScript] 자바스크립트 Template Literal (0) | 2023.05.10 |